It is the final countdown
SO this weeks challenge was to make a multi LED count down to some even. I chose my event to me a RGB-LED go through the entire spectrum of it's colors. So here is the code. I spliced a lot of the code from the example that our teacher gave us and then added my own. So here it is...
const int RED_PIN = 9;
const int GREEN_PIN = 11;
const int BLUE_PIN = 10;
int red_LED = 13;
int yellow_LED = 12;
int green_LED = 8;
int knob = A0;
int DISPLAY_TIME = 10;
void setup() {
pinMode(RED_PIN, OUTPUT);
pinMode(GREEN_PIN, OUTPUT);
pinMode(BLUE_PIN, OUTPUT);
pinMode(red_LED, OUTPUT);
pinMode(yellow_LED, OUTPUT);
pinMode(green_LED, OUTPUT);
}
void loop() {
//int displayTime = analogRead(knob);
digitalWrite(red_LED, HIGH);
delay(2500);
digitalWrite(red_LED, LOW);
digitalWrite(yellow_LED, HIGH);
delay(2500);
digitalWrite(yellow_LED, LOW);
digitalWrite(green_LED, HIGH);
delay(2500);
digitalWrite(green_LED, LOW);
//countDown();
showSpectrum();
}
void countDown()
{
// Red (turn just the red LED on):
digitalWrite(RED_PIN, HIGH);
digitalWrite(GREEN_PIN, LOW);
digitalWrite(BLUE_PIN, LOW);
delay(1000);
// Yellow
analogWrite(RED_PIN, 200);
analogWrite(GREEN_PIN, 55);
analogWrite(BLUE_PIN, 0);
delay(1000);
// Green (turn just the green LED on):
digitalWrite(RED_PIN, LOW);
digitalWrite(GREEN_PIN, HIGH);
digitalWrite(BLUE_PIN, LOW);
delay(1000);
// Off (all LEDs off):
digitalWrite(RED_PIN, LOW);
digitalWrite(GREEN_PIN, LOW);
digitalWrite(BLUE_PIN, LOW);
delay(1000);
}
void showSpectrum()
{
int x; // define an integer variable called "x"
// Now we'll use a for() loop to make x count from 0 to 767
// (Note that there's no semicolon after this line!
// That's because the for() loop will repeat the next
// "statement", which in this case is everything within
// the following brackets {} )
for (x = 0; x < 768; x++)
// Each time we loop (with a new value of x), do the following:
{
showRGB(x); // Call RGBspectrum() with our new x
delay(DISPLAY_TIME); // Delay for 10 ms (1/100th of a second)
// Off (all LEDs off):
}
digitalWrite(RED_PIN, LOW);
digitalWrite(GREEN_PIN, LOW);
digitalWrite(BLUE_PIN, LOW);
delay(1000);
}
void showRGB(int color)
{
int redIntensity;
int greenIntensity;
int blueIntensity;
// Here we'll use an "if / else" statement to determine which
// of the three (R,G,B) zones x falls into. Each of these zones
// spans 255 because analogWrite() wants a number from 0 to 255.
// In each of these zones, we'll calculate the brightness
// for each of the red, green, and blue LEDs within the RGB LED.
if (color <= 255) // zone 1
{
redIntensity = 255 - color; // red goes from on to off
greenIntensity = color; // green goes from off to on
blueIntensity = 0; // blue is always off
}
else if (color <= 511) // zone 2
{
redIntensity = 0; // red is always off
greenIntensity = 255 - (color - 256); // green on to off
blueIntensity = (color - 256); // blue off to on
}
else // color >= 512 // zone 3
{
redIntensity = (color - 512); // red off to on
greenIntensity = 0; // green is always off
blueIntensity = 255 - (color - 512); // blue on to off
}
// Now that the brightness values have been set, command the LED
// to those values
analogWrite(RED_PIN, redIntensity);
analogWrite(BLUE_PIN, blueIntensity);
analogWrite(GREEN_PIN, greenIntensity);
}
Here is the picture of my circuit.
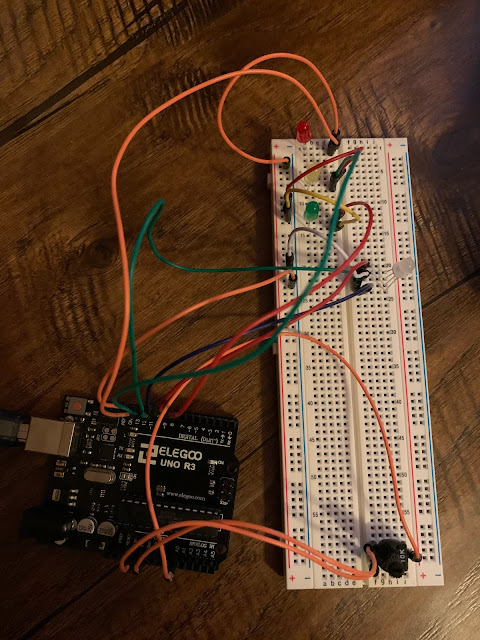
This is the video of how it worked and everything.
This weeks challenge was not all that difficult. I have learned a lot over the last three weeks and this was not that difficult for me to complete. I think being able to copy the code form some one else and splice it in made it a whole lot easier to finish this project and I know how to trouble shoot this type of circuit and code really quickly because of the last three weeks of experience. I think that if this had been the first project I would have given up then and not been able to do anything but I think I have learned quite a bit. So I guess what I am saying is that I didn't really remember much on how I did this circuit because I put it together pretty quickly because of my past experience and I hope that I just continue to learn and it becomes this easy for everything. I think that were you can find a program very similar to this is in my wife's diffuser. She has this oil diffuser that has and LED that makes that same light that my LED at the end made. I don't know where else you could see it but that was immediately apparent. Any ways happy making everyone.
const int RED_PIN = 9;
const int GREEN_PIN = 11;
const int BLUE_PIN = 10;
int red_LED = 13;
int yellow_LED = 12;
int green_LED = 8;
int knob = A0;
int DISPLAY_TIME = 10;
void setup() {
pinMode(RED_PIN, OUTPUT);
pinMode(GREEN_PIN, OUTPUT);
pinMode(BLUE_PIN, OUTPUT);
pinMode(red_LED, OUTPUT);
pinMode(yellow_LED, OUTPUT);
pinMode(green_LED, OUTPUT);
}
void loop() {
//int displayTime = analogRead(knob);
digitalWrite(red_LED, HIGH);
delay(2500);
digitalWrite(red_LED, LOW);
digitalWrite(yellow_LED, HIGH);
delay(2500);
digitalWrite(yellow_LED, LOW);
digitalWrite(green_LED, HIGH);
delay(2500);
digitalWrite(green_LED, LOW);
//countDown();
showSpectrum();
}
void countDown()
{
// Red (turn just the red LED on):
digitalWrite(RED_PIN, HIGH);
digitalWrite(GREEN_PIN, LOW);
digitalWrite(BLUE_PIN, LOW);
delay(1000);
// Yellow
analogWrite(RED_PIN, 200);
analogWrite(GREEN_PIN, 55);
analogWrite(BLUE_PIN, 0);
delay(1000);
// Green (turn just the green LED on):
digitalWrite(RED_PIN, LOW);
digitalWrite(GREEN_PIN, HIGH);
digitalWrite(BLUE_PIN, LOW);
delay(1000);
// Off (all LEDs off):
digitalWrite(RED_PIN, LOW);
digitalWrite(GREEN_PIN, LOW);
digitalWrite(BLUE_PIN, LOW);
delay(1000);
}
void showSpectrum()
{
int x; // define an integer variable called "x"
// Now we'll use a for() loop to make x count from 0 to 767
// (Note that there's no semicolon after this line!
// That's because the for() loop will repeat the next
// "statement", which in this case is everything within
// the following brackets {} )
for (x = 0; x < 768; x++)
// Each time we loop (with a new value of x), do the following:
{
showRGB(x); // Call RGBspectrum() with our new x
delay(DISPLAY_TIME); // Delay for 10 ms (1/100th of a second)
// Off (all LEDs off):
}
digitalWrite(RED_PIN, LOW);
digitalWrite(GREEN_PIN, LOW);
digitalWrite(BLUE_PIN, LOW);
delay(1000);
}
void showRGB(int color)
{
int redIntensity;
int greenIntensity;
int blueIntensity;
// Here we'll use an "if / else" statement to determine which
// of the three (R,G,B) zones x falls into. Each of these zones
// spans 255 because analogWrite() wants a number from 0 to 255.
// In each of these zones, we'll calculate the brightness
// for each of the red, green, and blue LEDs within the RGB LED.
if (color <= 255) // zone 1
{
redIntensity = 255 - color; // red goes from on to off
greenIntensity = color; // green goes from off to on
blueIntensity = 0; // blue is always off
}
else if (color <= 511) // zone 2
{
redIntensity = 0; // red is always off
greenIntensity = 255 - (color - 256); // green on to off
blueIntensity = (color - 256); // blue off to on
}
else // color >= 512 // zone 3
{
redIntensity = (color - 512); // red off to on
greenIntensity = 0; // green is always off
blueIntensity = 255 - (color - 512); // blue on to off
}
// Now that the brightness values have been set, command the LED
// to those values
analogWrite(RED_PIN, redIntensity);
analogWrite(BLUE_PIN, blueIntensity);
analogWrite(GREEN_PIN, greenIntensity);
}
Here is the picture of my circuit.
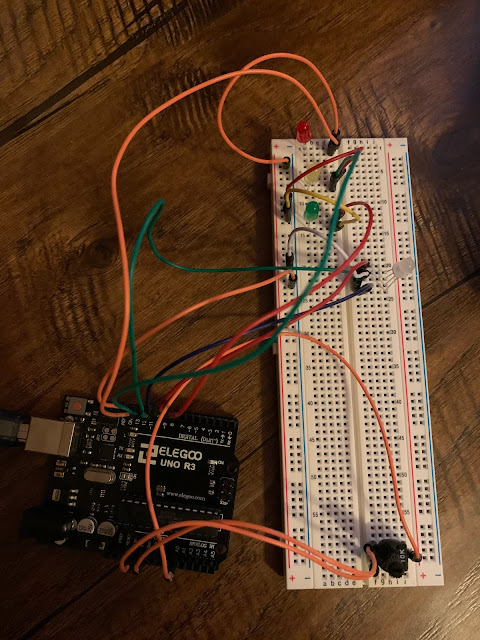
This is the video of how it worked and everything.
This weeks challenge was not all that difficult. I have learned a lot over the last three weeks and this was not that difficult for me to complete. I think being able to copy the code form some one else and splice it in made it a whole lot easier to finish this project and I know how to trouble shoot this type of circuit and code really quickly because of the last three weeks of experience. I think that if this had been the first project I would have given up then and not been able to do anything but I think I have learned quite a bit. So I guess what I am saying is that I didn't really remember much on how I did this circuit because I put it together pretty quickly because of my past experience and I hope that I just continue to learn and it becomes this easy for everything. I think that were you can find a program very similar to this is in my wife's diffuser. She has this oil diffuser that has and LED that makes that same light that my LED at the end made. I don't know where else you could see it but that was immediately apparent. Any ways happy making everyone.
Comments
Post a Comment